Web Development Bootcamp
Free
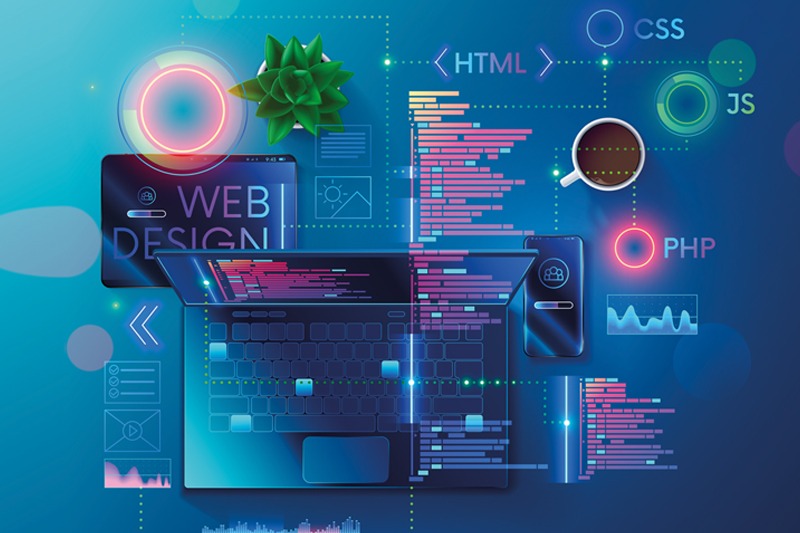
What you’ll learn
-
Be able to build ANY website you want.
-
Craft a portfolio of websites to apply for junior developer jobs.
-
Build fully-fledged websites and web apps for your startup or business.
-
Work as a freelance web developer.
-
Master backend development with Node
-
Master frontend development with React
-
Learn the latest frameworks and technologies, including Javascript ES6, Bootstrap 4, MongoDB.
For customized courses contact the administrator.
To book a trial course follow the link
Course Features
- Lectures 290
- Quizzes 0
- Duration 50 hours
- Skill level All levels
- Language English
- Students 1
- Certificate No
- Assessments Yes
-
Introduction
-
Introduction to HTML
-
Intermediate HTML
-
Introduction to CSS
-
Intermediate CSS
- What We’ll Make – Stylised Personal Site
- What Are Favicons?
- HTML Divs
- The Box Model of Website Styling
- CSS Display Property
- CSS Static and Relative Positioning
- Absolute positioning
- The Dark Art of Centering Elements with CSS
- Font Styling in Our Personal Site
- Adding Content to Our Website
- CSS Sizing
- CSS Font Property Challenge Solutions
- CSS Float and Clear
- Stylised Personal Site Solution Walkthrough
-
Introduction to Bootstrap 4
- What is Bootstrap?
- Installing Bootstrap
- Web Design 101 – Wireframing
- The Bootstrap Navigation Bar
- What We’ll Make : TinDog
- Setting Up Our New Project
- Bootstrap Grid Layout System
- Adding Grid Layouts to Our Website
- Bootstrap Containers
- Bootstrap Buttons & Font Awesome
- Styling Our Website Challenges and Solutions
- Solution to Bootstrap Challenge 1
-
Intermediate Bootstrap
- The Bootstrap Carousel Part 1
- The Bootstrap Carousel Part 2
- Bootstrap Cards
- The CSS Z-Index and Stacking Order
- Media Query Breakpoints
- Bootstrap Challenge 2 Solution
- How to become a Better Programmer – Code Refactoring
- Put it into Practice – Refactor our Website Part 1
- Advanced CSS – Combining Selectors
- Refactoring our Website Part 2
- Advanced CSS – Selector Priority
- Completing the Website
-
Web Design School
-
Introduction to Javascript ES6
- Introduction to Javascript
- Javascript Alerts – Adding Behaviour to Websites
- Data Types
- Javascript Variables
- Javascript Variables Exercise Start
- Javascript Variables Exercise Solution
- Naming and Naming Conventions for Javascript Variables
- String Concatenation
- String Lengths and Retrieving the Number of Characters
- Slicing and Extracting Parts of a String
- Challenge: Changing Casing in Text
- Challenge: Changing String Casing Solution
- Basic Arithmetic and the Modulo Operator in Javascript
- Increment and Decrement Expressions
- Functions Part 1: Creating and Calling Functions
- Functions Part 1 Challenge – The Karel Robot
- Functions Part 2: Parameters and Arguments
- Life in Weeks Solution
- Functions Part 3: Outputs & Return Values
- Challenge: Create a BMI Calculator
- Challenge: BMI Calculator Solution
-
Intermediate Javascript
- Random Number Generation in Javascript: Building a Love Calculator
- Control Statements: Using If-Else Conditionals & Logic
- Comparators and Equality
- Combining Comparators
- Introducing the Leap Year Code Challenge
- Leap Year Solution
- Collections: Working with Javascript Arrays
- Adding Elements and Intermediate Array Techniques
- Who’s Buying Lunch Solution
- Control Statements: While Loops
- Control Statements: For Loops
- Introducing the Fibonacci Code Challenge
- Fibonacci Solution
-
Document Object Model (DOM)
- Adding Javascript to Websites
- Introduction to the Document Object Model (DOM)
- Selecting HTML Elements with Javascript
- Manipulating and Changing Styles of HTML Elements with Javascript
- The Separation of Concerns: Structure vs Style vs Behaviour
- Text Manipulation and the Text Content Property
- Manipulating HTML Element Attributes
-
Advanced Javascript and DOM Manipulation
- Drum Kit
- Adding Event Listeners to a Button
- Higher Order Functions and Passing Functions as Arguments
- How to Play Sounds on a Website
- A Deeper Understanding of Javascript Objects
- How to Use Switch Statements in Javascript
- Objects, their Methods and the Dot Notation
- Using Keyboard Event Listeners to Check for Key Presses
- Understanding Callbacks and How to Respond to Events
- Adding Animation to Websites
-
jQuery
- What is jQuery?
- How to Incorporate jQuery into Websites
- How Minification Works to Reduce File Size
- Selecting Elements with jQuery
- Manipulating Styles with jQuery
- Manipulating Text with jQuery
- Manipulating Attributes with jQuery
- Adding Event Listeners with jQuery
- Adding and Removing Elements with jQuery
- Website Animations with jQuery
-
The Unix Command Line
-
Backend Web Development
-
Node.js
-
Express.js with Node.js
- What is Express?
- Creating Our First Server with Express
- Handling Requests and Responses: the GET Request
- Nodemon Installation
- Understanding and Working with Routes
- What We’ll Make: A Calculator
- Calculator Setup: Challenge and Solution
- Responding to Requests with HTML Files
- Processing Post Requests with Body Parser
- BMI Routing Challenge
- Solution to the BMI Routing Challenge
-
APIs - Application Programming Interfaces
- Why Do We Need APIs?
- API Endpoints, Paths and Parameters.
- API Authentication and Postman
- What is JSON?
- Making GET Requests with the Node HTTPS Module
- How to Parse JSON
- Using Express to Render a Website with Live API Data
- Using Body Parser to Parse POST Requests to the Server
- The Mailchimp API – What You’ll Make
- Setting Up the Sign Up Page
- Posting Data to Mailchimp’s Servers via their API
- Adding Success and Failure Pages
- Deploying Your Server with Heroku
-
Git, Github and Version Control
-
EJS
- A To Do list
- Templates? Why Do We Need Templates?
- Creating Your First EJS Templates
- Running Code Inside the EJS Template
- Passing Data from Your Webpage to Your Server
- The Concept of Scope in the Context of Javascript
- Adding Pre-Made CSS Stylesheets to Your Website
- Understanding Templating vs. Layouts
- Understanding Node Module Exports: How to Pass Functions and Data between Files
-
Additional Excercise - Blog Website
-
Databases
-
SQL
-
MongoDB
- Installing MongoDB on Mac
- Installing MongoDB on Windows
- MongoDB CRUD Operations in the Shell: Create
- MongoDB CRUD Operations in the Shell: Reading & Queries
- MongoDB CRUD Operations in the Shell: Update
- MongoDB CRUD Operations in the Shell: Delete
- Relationships in MongoDB
- Working with The Native MongoDB Driver
-
Mongoose
-
Putting Everything Together
- Let’s take the ToDoList Project to the Next Level and Connect it with Mongoose
- Rendering Database Items in the ToDoList App
- Adding New Items to our ToDoList Database
- Deleting Items from our ToDoList Database
- Creating Custom Lists using Express Route Parameters
- Adding New Items to the Custom ToDo Lists
- Revisiting Lodash and Deleting Items from Custom ToDo Lists
-
Deploying your Web Application
-
RESTful API
-
Authentication and Security
- Introduction to Authentication
- Getting Set Up
- Level 1 – Register Users with Username and Password
- Level 2 – Database Encryption
- Using Environment Variables to Keep Secrets Safe
- Level 3 – Hashing Passwords
- Hacking 101 ☣️
- Level 4 – Salting and Hashing Passwords with bcrypt
- What are Cookies and Sessions?
- Using Passport.js to Add Cookies and Sessions
- Level 6 – OAuth 2.0 & How to Implement Sign In with Google
- Finishing Up the App – Letting Users Submit Secrets
-
React.js
- What is React?
- What we will make in this React module
- Introduction to Code Sandbox and the Structure of the Module
- Introduction to JSX and Babel
- JSX Code Practice
- Javascript Expressions in JSX & ES6 Template Literals
- Javascript Expressions in JSX Practice
- JSX Attributes & Styling React Elements
- Inline Styling for React Elements
- React Styling Practice
- React Components
- React Components Practice
- Javascript ES6 – Import, Export and Modules
- Javascript ES6 Import, Export and Modules Practice
- [Windows] Local Environment Setup for React Development
- [Mac] Local Environment Setup for React Development
- Keeper App Project – Part 1 Challenge
- Keeper App Part 1 Solution
- React Props
- React Props Practice
- React DevTools
- Mapping Data to Components
- Mapping Data to Components Practice
- Javascript ES6 Map/Filter/Reduce
- Javascript ES6 Arrow functions
- Keeper App Project – Part 2
- React Conditional Rendering with the Ternary Operator & AND Operator
- Conditional Rendering Practice
- State in React – Declarative vs. Imperative Programming
- React Hooks – useState
- useState Hook Practice
- Javascript ES6 Object & Array Destructuring
- Javascript ES6 Destructuring Challenge Solution
- Event Handling in React
- React Forms
- Class Components vs. Functional Components
- Changing Complex State
- Changing Complex State Practice
- Javascript ES6 Spread Operator
- Javascript ES6 Spread Operator Practice
- Managing a Component Tree
- Managing a Component Tree Practice
- Keeper App Project – Part 3
- React Dependencies & Styling the Keeper App
-
Conclusion